Regular expressions, often abbreviated as regex, are powerful tools for pattern matching and text manipulation. Whether you’re a beginner or an experienced programmer, understanding regular expressions can greatly enhance your ability to search, validate, and extract data from text. In this comprehensive guide, we’ll take you through the fundamentals of regular expressions, providing you with the knowledge and skills to write like a pro. So, let’s dive in and unravel the mysteries of regular expressions together!
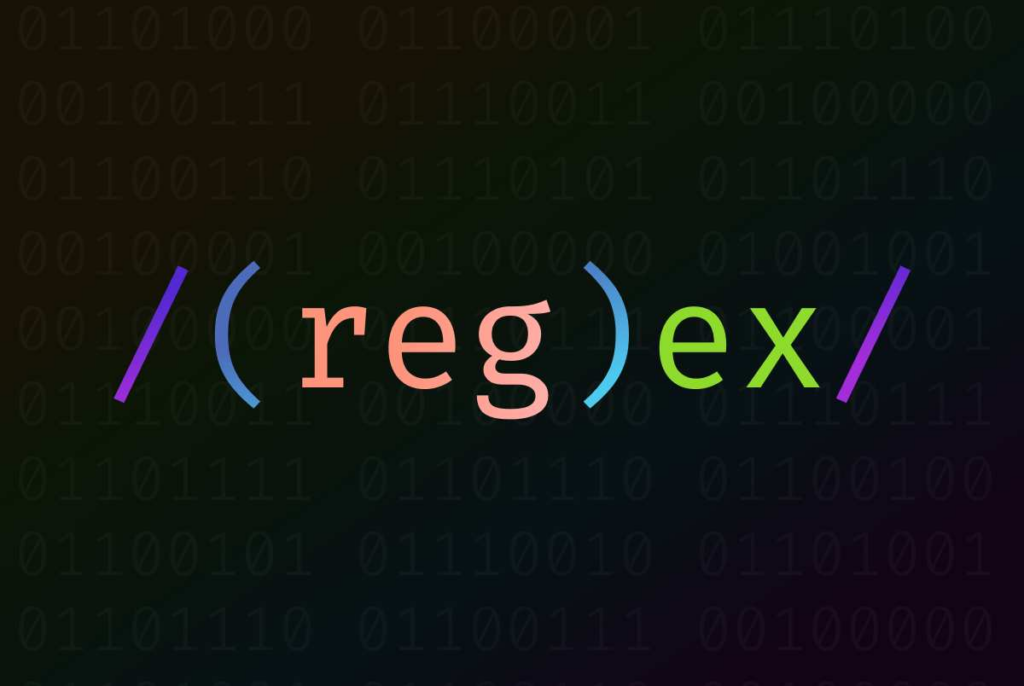
What Are Regular Expressions?
Regular expressions are sequences of characters that define search patterns. They provide a concise and flexible way to match, validate, and manipulate text. Regular expressions are supported by many programming languages, text editors, and command-line tools, making them a versatile tool in a developer’s arsenal.
Basic Syntax and Usage
To start using regular expressions, you need to understand the basic syntax and usage. Regular expressions consist of literal characters, metacharacters, and special sequences. They are often enclosed in forward slashes (/) to differentiate them from normal text. Let’s explore some examples:
/hello/
matches the word “hello” in a text.
/[aeiou]/
matches any vowel character.
/[0-9]/
matches any digit character.
/[A-Za-z]/
matches any uppercase or lowercase letter.
Matching Literal Characters
When you want to match literal characters, you can simply include them in the regular expression. For example, the regular expression /cat/
will match the word “cat” in a text. However, it is important to note that regular expressions are case-sensitive by default. To perform a case-insensitive match, you can use the /i
flag at the end of the expression, like this: /cat/i
.
Metacharacters and Special Sequences
Metacharacters are special characters with a predefined meaning in regular expressions. They allow you to create more complex patterns. Some common metacharacters include:
.
(dot): Matches any character except a newline.
*
(asterisk): Matches zero or more occurrences of the preceding character or group.
+
(plus): Matches one or more occurrences of the preceding character or group.
?
(question mark): Matches zero or one occurrence of the preceding character or group.
|
(pipe): Matches either the expression before or after the pipe.
()
(parentheses): Groups characters or expressions together.
Special sequences are predefined patterns that match specific types of characters or character classes. Some useful special sequences include:
\d
: Matches any digit character.
\w
: Matches any alphanumeric character (word character).
\s
: Matches any whitespace character.
\b
: Matches a word boundary.
Quantifiers and Repetition
Quantifiers allow you to specify the number of occurrences that should be matched. They follow a character or group and control how many times it can appear. Some common quantifiers include:
*
(asterisk): Matches zero or more occurrences.
+
(plus): Matches one or more occurrences.
?
(question mark): Matches zero or one occurrence.
{n}
: Matches exactly n occurrences.
{n,}
: Matches n or more occurrences.
{n,m}
: Matches between n and m occurrences.
For example, the regular expression /a+/
will match one or more consecutive “a” characters, such as “aa,” “aaa,” and so on.
Anchors and Boundaries
Anchors and boundaries are special characters that match specific positions in the text. They are useful for ensuring that a pattern occurs at a specific location. Some common anchors and boundaries include:
^
(caret): Matches the start of a line or string.
$
(dollar sign): Matches the end of a line or string.
\b
: Matches a word boundary.
For example, the regular expression /^cat/
will match the word “cat” only if it appears at the beginning of a line or string.
Character Classes and Alternation
Character classes allow you to specify a set of characters that can be matched. They are enclosed in square brackets ([]). For example, the regular expression /[aeiou]/
matches any vowel character. You can also use ranges to match a range of characters, such as /[a-z]/
for any lowercase letter.
Alternation is denoted by the pipe character (|) and allows you to match either the expression before or after the pipe. For example, the regular expression /apple|orange/
will match either “apple” or “orange” in a text.
Grouping and Capturing
Grouping allows you to treat multiple characters or expressions as a single unit. This is useful when you want to apply quantifiers or alternation to a group of characters. Grouping is achieved using parentheses. For example, the regular expression /(abc)+/
matches one or more occurrences of the sequence “ABC.”
See it in action at regex101: https://regex101.com/r/eU5F4n/1
Capturing allows you to extract specific parts of a match. When you use parentheses around a group, you can refer to the captured content using backreferences. Backreferences are denoted by a backslash followed by a number (\1, \2, etc.). For example, the regular expression /(\d+)-(\w+)/
captures a number followed by a hyphen and a word.
See it in action at regex101: https://regex101.com/r/GQO7c7/1
Lookaheads and Lookbehinds
Lookaheads and lookbehinds are zero-width assertions that match a specific pattern without including it in the final match. Lookaheads are denoted by (?=pattern)
for positive lookaheads and (?!pattern)
for negative lookaheads. Lookbehinds are denoted by (?<=pattern)
for positive lookbehinds and (?<!pattern)
for negative lookbehinds.
These assertions are useful when you want to match a pattern only if it is followed or preceded by another pattern. For example, the regular expression /\d+(?= dollars)/
matches a number only if it is followed by the word “dollars.”
See it in action at regex101: https://regex101.com/r/IF92wM/1
Backreferences
Backreferences allow you to match a previously captured group within the same regular expression. They are denoted by a backslash followed by a number (\1, \2, etc.). Backreferences are useful when you want to ensure that a repeated pattern occurs multiple times.
For example, the regular expression /(\w+)\s+\1/
matches a word followed by one or more whitespace characters and then the same word again.
See it in action at regex101: https://regex101.com/r/IfhAYv/1
Greedy vs. Lazy Matching
By default, regular expressions use greedy matching, which means they try to match as much as possible. However, there are cases where you may want to use lazy matching, which matches as little as possible. You can make a quantifier lazy by appending a question mark (?).
For example, the regular expression /a.+?b/
will match the shortest possible sequence between “a” and “b” in a text.
Escaping Special Characters
In regular expressions, some characters have special meanings and need to be escaped if you want to match them literally. These characters include [ ] { } ( ) . * + ? ^ $ \ |
. To escape a special character, precede it with a backslash ().
For example, to match the literal dot character “.”, you need to use the regular expression /\./
.
Practical Examples
Validating Email Addresses
You can use regex to check if an email address is valid or not. For example, the pattern ^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$
can be used to validate email addresses.
See it in action at regex101: https://regex101.com/r/C1XcvY/1
Extracting URLs from Text
Regex can be used to extract URLs from a block of text. For instance, the pattern https?://\S+
can match URLs starting with “http://” or “https://”, followed by any non-whitespace characters.
See it in action at regex101: https://regex101.com/r/nyUWjP/1
Finding and Replacing Text:
Find and replace specific patterns of text. For example, you can use the pattern s/old/new/g
to replace all occurrences of “old” with “new” in a text.
Validating Phone Numbers
Can be used to validate phone numbers in a specific format. For instance, the pattern ^\d{3}-\d{3}-\d{4}$
can match phone numbers in the format “###-###-####”.
See it in action at regex101: https://regex101.com/r/UyHgU1/1
Parsing CSV Data
Regex can be useful for parsing CSV (Comma-Separated Values) data. You can define a pattern that matches the structure of a CSV row and use it to extract individual values
Extracting Hashtags
Hashtags can be extracted from social media posts or text. For example, the pattern #\w+
can match hashtags starting with a “#” followed by one or more word characters.
See it in action at regex101: https://regex101.com/r/79a4lB/1
Removing Special Characters
Regex can be used to remove or replace special characters in a text. For instance, the pattern [^a-zA-Z0-9\s]
can match any character that is not a letter, digit, or whitespace, which can then be removed or replaced.
Validating Credit Card Numbers
Regex can be employed to validate credit card numbers based on their format. There are different patterns for different card types, such as Visa, Mastercard, or American Express.
Extracting Dates
Dates can be extracted from text in various formats. For example, the pattern (\d{2})/(\d{2})/(\d{4})
can match dates in the format “DD/MM/YYYY” and extract the day, month, and year.
See it in action at regex101: https://regex101.com/r/Xumpcx/1
Tokenizing Text
Can be used to split text into tokens based on specific patterns. For instance, you can use the pattern \b\w+\b
to match individual words in a sentence and tokenize the text accordingly.
See it in action at regex101: https://regex101.com/r/eKfndW/1
Tips and Best Practices
When working with regular expressions, here are some tips and best practices to keep in mind:
Keep it simple: Start with simple patterns and gradually build upon them as needed.
Test incrementally: Test your regular expressions on small inputs before applying them to larger datasets.
Use online tools: There are many online tools available that allow you to test and debug regular expressions. We use regex101 throughout the article to show you certain expressions in action.
Comment your patterns: Regular expressions can be complex, so adding comments can help you and others understand the intention of your patterns.
Optimize when necessary: Regular expressions can be resource-intensive, so optimize them if they become a performance bottleneck in your application.
Common Mistakes to Avoid
Regular expressions can be tricky, and there are some common mistakes that beginners make. Here are a few to avoid:
Forgetting to escape special characters: If you want to match special characters literally, remember to escape them with a backslash.
Overusing or misusing quantifiers: Be careful when using quantifiers, as they can lead to unexpected matches if not used correctly.
Not considering edge cases: Regular expressions should account for various edge cases to ensure accurate matching and validation.
Writing overly complex patterns: While regular expressions are powerful, it’s important to strike a balance between complexity and readability.
Not testing thoroughly: Always test your regular expressions against a variety of test cases to ensure they work as intended.
Debugging and Testing Tools
Debugging and testing regular expressions can be challenging, especially for complex patterns. Fortunately, there are several tools available that can assist you in this process:
- Regex101: An online regex tester and debugger that provides real-time matching and explanation of regular expressions.
- RegExr: A web-based tool that allows you to test and learn regular expressions interactively.
- RegexBuddy: A commercial tool that provides a comprehensive regex debugger and builder.
Regex FAQs
How Can Regular Expressions Improve Efficiency?
Regular expressions can improve efficiency by allowing you to perform complex pattern-matching and text manipulation tasks in a concise and efficient manner. Instead of writing lengthy code to search and extract specific patterns, regular expressions provide a compact solution.
Are Regular Expressions Case Sensitive?
By default, regular expressions are case-sensitive. This means that uppercase and lowercase letters are treated as distinct characters. To perform a case-insensitive match, you can use the /i
flag at the end of the expression, as mentioned earlier.
Can Regular Expressions Match Across Multiple Lines?
By default, regular expressions match single lines of text. However, some programming languages and tools provide flags or options to enable multiline matching. For example, in Python, the re.MULTILINE
flag allows regular expressions to match across multiple lines.
What Are the Limitations of Regular Expressions?
Although powerful, regular expressions have certain limitations. They are not suitable for parsing complex nested structures, such as HTML or XML, where a dedicated parser is recommended. Regular expressions can also be difficult to read and understand for complex patterns, making them prone to errors.
Are Regular Expressions Language-Specific?
While regular expression flavors can vary slightly between programming languages and libraries, there are common features and syntaxes used across most implementations. Here are some commonly supported features and flavors in regex:
POSIX Basic and Extended Regular Expressions (BRE and ERE): These are traditional regex flavors supported by POSIX-compliant systems. BRE uses basic metacharacters, while ERE extends the syntax with additional metacharacters and features.
Perl-Compatible Regular Expressions (PCRE): PCRE is widely used in languages like Perl, PHP, and Python. It provides advanced features such as lookahead and look-behind assertions, named capturing groups, and backreferences.
JavaScript Regular Expressions: JavaScript uses its own flavor of regex, which is similar to PCRE but with some variations. It supports the most common regex features but lacks some advanced capabilities like look-behind assertions.
.NET Regular Expressions: The .NET framework provides its own regex flavor, which is similar to PCRE but with some additional features. It supports advanced options like balancing groups and atomic groups.
Java Regular Expressions: Java has built-in support for regular expressions using the java.util.regex package. It is similar to the syntax used in Perl and PCRE but has a few differences.
Python Regular Expressions: Python has a built-in regex module called “re” that supports a syntax similar to PCRE. It offers a wide range of regex features, including lookahead and look-behind assertions, named capturing groups, and more.
Conclusion
Regular expressions are a valuable tool for pattern matching and text manipulation. With a solid understanding of their syntax and features, you can write powerful and efficient regular expressions to search, validate, and extract specific patterns from text. Whether you’re a beginner or an experienced developer, mastering regular expressions can greatly enhance your text-processing capabilities.
So, don’t be intimidated by the seemingly cryptic syntax. Regular expressions for dummies can be learned and mastered with practice and patience. With this guide, you’re well on your way to becoming a pro at writing regular expressions!